Physical Computing - Infrared Motion Sensor, Attitude Sensor MPU6050, Bluetooth, and Wi-Fi
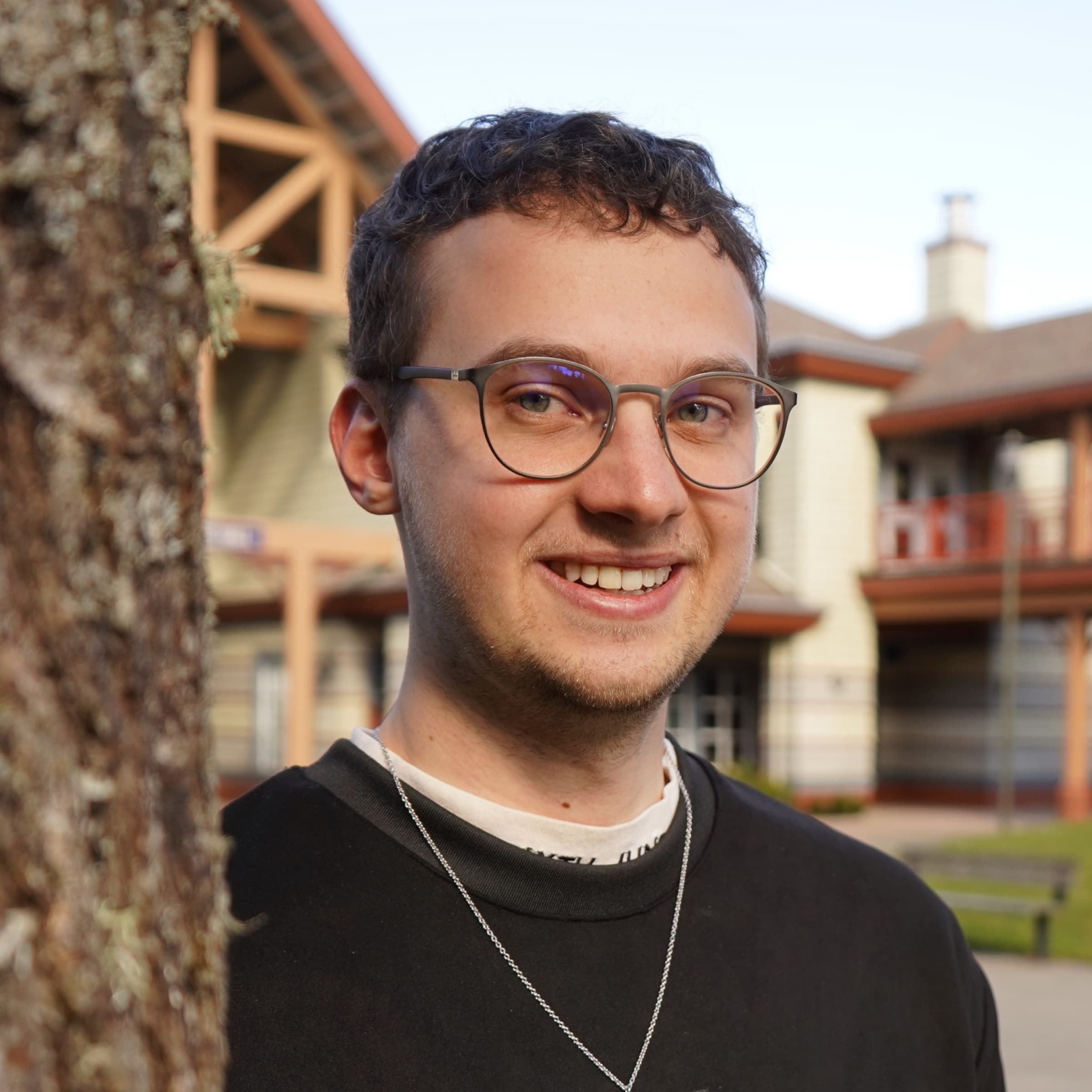
Adam Boucek
|November 14th 2023
In this lab, we will continue with another set of projects you can create with ESP32-WROVER. Instructions are given by Andrew J. Park, Ph.D..
We will use Freenove Ultimate Starter Kit for ESP32-WROVER which you can buy on Amazon. How to set up the kit and start uploading code you can find on Freenove.
If these projects seem too challenging, you can try to do the Freenove tutorials. See them in the tutorials section ↓.
Project 1 - Burglar alarm
Date: 11/13/23
Instructions:
Design and construct a burglar alarm system with an HC SR501 PIR sensor, a buzzer, an RGB LED, a servo or stepper motor, and other necessary electronic components. Attach a paper hand to the motor. When no one is around the HC SR501 sensor, the RGB LED shows a dim light with a calming colour (like a night light). But when a burglar approaches the PIR sensor, the buzzer makes a siren alarm sound effect, the RGB LED flashes rapidly with changing random colours, and the paper hand waves rapidly. Capture your settings and working circuit in photos and videos (the videos should include sound).
Parts:
- ESP32-WROVER x1
- GPIO Extension Board x1 (optional)
- Breadboard x1
- Jumper M/M x14
- HC SR501 x1
- NPN transistor x1
- Passive Buzzer x7
- Resistor 1kΩ x1
- Resistor 220Ω x3
- Servo Motor x1
- RGB LED x1
Burglar alarm Schema:
Burglar alarm Breadboard View:
Source Code:
project-1.py
from machine import Pin,PWM import time import math import _thread from random import randint ############################################################# # Used code from Freenove Ultimate kit ESP32 tutorial 18.1 ############################################################# class myServo(object): def __init__(self, pin: int = 15, hz: int = 50): self._servo = PWM(Pin(pin), hz) def myServoWriteDuty(self, duty): if duty <= 26: duty = 26 if duty >= 128: duty = 128 self._servo.duty(duty) def myServoWriteAngle(self, pos): if pos <= 0: pos = 0 if pos >= 180: pos = 180 pos_buffer = (pos/180)*(128-26) self._servo.duty(int(pos_buffer)+26) def myServoWriteTime(self, us): if us <= 500: us = 500 if us >= 2500: us = 2500 pos_buffer = (1024*us)/20000 self._servo.duty(int(pos_buffer)) def deinit(self): self._servo.deinit() ############################################################# # Project code ############################################################# PI=3.14 # init pins RGBpins = [15, 2, 0] servo = myServo(4) # set servo pin servo.myServoWriteAngle(0) # Set Servo Angle pwm0 = PWM(Pin(RGBpins[0]), 10000) # Red pwm1 = PWM(Pin(RGBpins[1]), 10000) # Green pwm2 = PWM(Pin(RGBpins[2]), 10000) # Blue passiveBuzzer=PWM(Pin(13),2000) sensorPin=Pin(14,Pin.IN) def alert(): passiveBuzzer.init() for x in range(0,36): sinVal=math.sin(x*10*PI/180) toneVal=2000+int(sinVal*500) passiveBuzzer.freq(toneVal) time.sleep_ms(10) passiveBuzzer.deinit() def waveHand(): for i in range(0, 45, 1): # servo goes up servo.myServoWriteAngle(i) time.sleep_ms(15) for i in range(45, 0, -1): # servo goes down servo.myServoWriteAngle(i) time.sleep_ms(15) def setColor(r,g,b): pwm0.duty(1023-r) pwm1.duty(1023-g) pwm2.duty(1023-b) def alarmLED(): for x in range(0, 10): red = randint(0,1023) green = randint(0,1023) blue = randint(0,1023) setColor(red,green,blue) time.sleep_ms(200) #brightness :0-255 brightness=10 colors=[[brightness,0,0], #red [0,brightness,0], #green [0,0,brightness], #blue [brightness,brightness,brightness], #white [0,0,0]] #close try: while True: print(sensorPin.value()) passiveBuzzer.deinit() setColor(500,0,0) if sensorPin.value(): passiveBuzzer.init() second_thread = _thread.start_new_thread(alert, ()) third_thread = _thread.start_new_thread(alarmLED, ()) waveHand() time.sleep_ms(200) time.sleep_ms(1000) except: pwm0.deinit() pwm1.deinit() pwm2.deinit()
Youtube video ↓ (click on it)
Project 2 - Fall Detector
Date: 11/13/23
Instructions:
Design and construct a circuit with an MPU6050, a buzzer, an LCD1602, and other necessary electronic components. This circuit is to help an older adult detect his/her balance and mobility. Assume that this circuit is attached to the user. When the user suddenly falls down forward, backward, to the left, or right, the circuit makes a distinctive sound effect for each direction, and the LCD1602 displays the appropriate message such as “You fell down forward” or “You fell down to the left.” Capture your settings and working circuit in photos and videos (the videos should include sound). In your video(s), simulate the falling downs in the four different directions and show the messages in the LCD1602 display.
Parts:
- ESP32-WROVER x1
- GPIO Extension Board x1 (optional)
- Breadboard x1
- LCD 1602 x1
- MPU6050 x1
- Jumper M/M x12
- NPN transistor x1
- Passive Buzzer x7
- Resistor 1kΩ x2
Fall Detector schema:
Fall Detector Breadboard View:
Source Code:
project-2.py
from machine import I2C,Pin, PWM from lcd import I2C, I2cLcd import time import math from mpu import MPU6050 PI=3.14 passiveBuzzer=PWM(Pin(13),2000) i2c = I2C(scl=Pin(33), sda=Pin(32), freq=400000) devices = i2c.scan() if len(devices) == 0: print("No i2c device !") else: for device in devices: print("I2C addr: "+hex(device)) lcd = I2cLcd(i2c, device, 2, 16) lcd.putstr("Made by \nAdam Boucek") mpu=MPU6050(14,12) #attach the IIC pin(sclpin,sdapin) mpu.MPU_Init() #initialize the MPU6050 G = 9.8 time.sleep_ms(1000)#waiting for MPU6050 to work steadily def alert(function,speed=180): if function=="sin": for x in range(0,36): sinVal=math.sin(x*10*PI/speed) toneVal=2000+int(sinVal*500) passiveBuzzer.freq(toneVal) time.sleep_ms(10) else: for x in range(0,36): sinVal=math.cos(x*10*PI/speed) toneVal=2000+int(sinVal*500) passiveBuzzer.freq(toneVal) time.sleep_ms(10) try: while True: passiveBuzzer.init() accel=mpu.MPU_Get_Accelerometer()#gain the values of Acceleration if(accel[0] < -15000 and accel[2] < -100): # right alert("sin") lcd.putstr("You fell down right") if(accel[0] < 15000 and accel[2] < -100): # left lcd.putstr("You fell down left") alert("cos") if(accel[1] < -15000): # forward lcd.putstr("You fell down forward") alert("sin",90) if(accel[1] < 15000): # back lcd.putstr("You fell down back") alert("cos",90) passiveBuzzer.deinit() time.sleep_ms(1000) except: pass
Youtube video ↓ (click on it)
Tutorials
Tutorials were done in class.