Physical Computing - Ultrasonic Ranging, Matrix Keypad, Infrared Remote, and Hygrothermograph DHT11
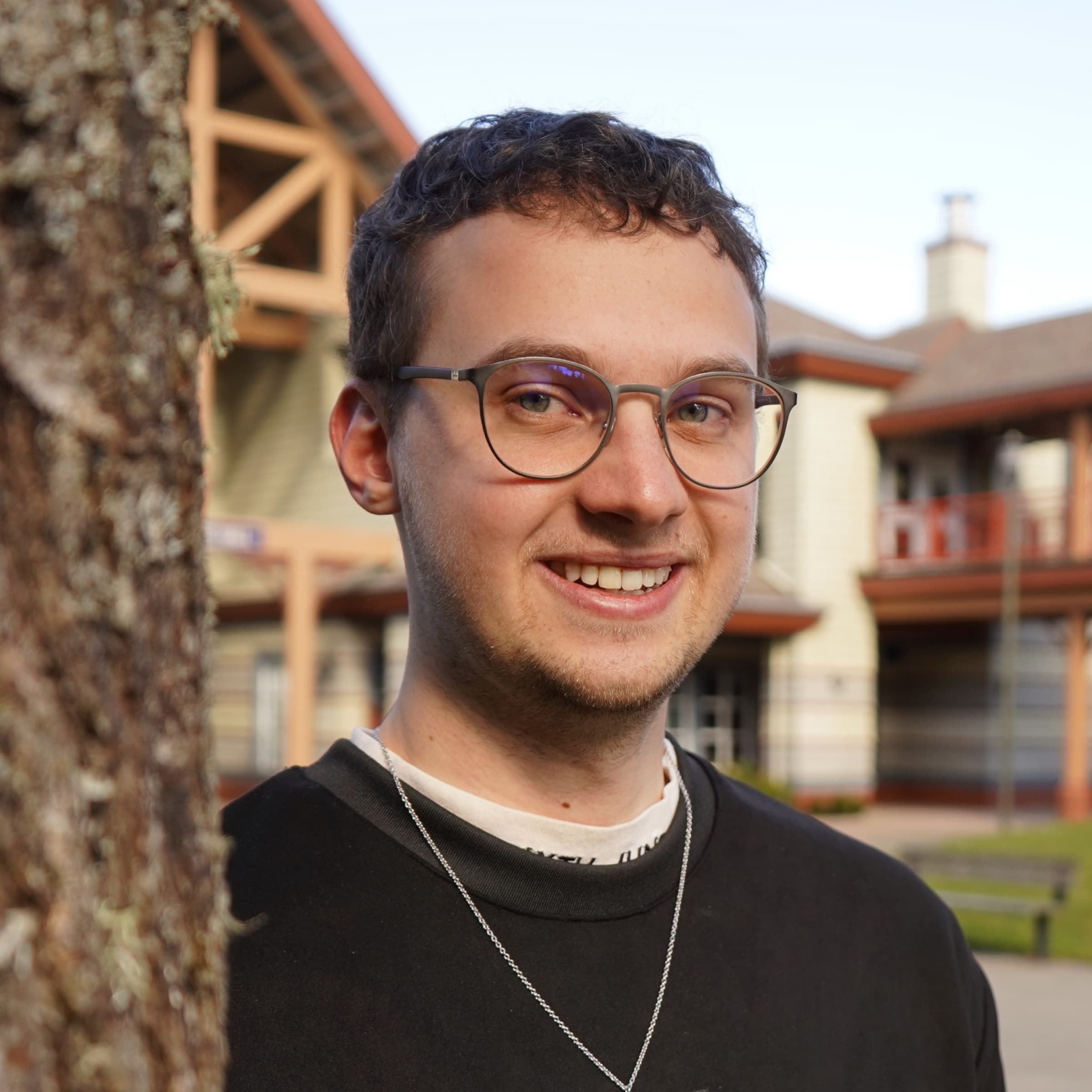
Adam Boucek
|November 2nd 2023
In this lab, we will continue with another set of projects you can create with ESP32-WROVER. Instructions are given by Andrew J. Park, Ph.D..
We will use Freenove Ultimate Starter Kit for ESP32-WROVER which you can buy on Amazon. How to set up the kit and start uploading code you can find on Freenove.
If these projects seem too challenging you can try to do the Freenove tutorials. See them in the tutorials section ↓.
Project 1 - Ultrasonic Ranging, a passive buzzer, an LED
Date: 11/2/23
Instructions:
Design and construct a circuit with an ultrasonic ranging module (HC-SR04), a buzzer, an LED bar (or multiple LEDs), and other necessary electronic components. When an object gets close to the HCSR04, the circuit increases the number of lit LEDs and plays higher tones (i.e., (low) Do-Re-Mi-Fa-SoLa-Ti-Do (high)). When an object goes away from the HC-SR04, the circuit decreases the number of lit LEDs and plays lower tones.
Parts:
- ESP32-WROVER x1
- GPIO Extension Board x1 (optional)
- Breadboard x1
- Jumper M/M x9
- HC-SR04 x1
- NPN transistor x1
- Passive Buzzer x7
- Resistor 1kΩ x2
- LED eed x1
Ultrasonic Ranging, a passive buzzer, an LED Schema:
Ultrasonic Ranging, a passive buzzer, an LED Breadboard View:
Source Code:
project-1.py
from machine import Pin,PWM import time # init pins trigPin=Pin(13,Pin.OUT,0) echoPin=Pin(14,Pin.IN,0) ledPin=PWM(Pin(25),30000) buzzerPin=PWM(Pin(18), 2000) ############################################################# # used a class from Freenove Ultimate kit ESP32 tutorial 21.1 ############################################################# soundVelocity=340 distance=0 def getSonar(): trigPin.value(1) time.sleep_us(10) trigPin.value(0) while not echoPin.value(): pass pingStart=time.ticks_us() while echoPin.value(): pass pingStop=time.ticks_us() pingTime=time.ticks_diff(pingStop,pingStart) distance=pingTime*soundVelocity//2//30000 return int(distance) # used from https://github.com/hibit-dev/buzzer/blob/master/lib/pitches.zip NOTES = { "B0": 31, "C1": 33, "CS1": 35, "D1": 37, "DS1": 39, "E1": 41, "F1": 44, "FS1": 46, "G1": 49, "GS1": 52, "A1": 55, "AS1": 58, "B1": 62, "C2": 65, "CS2": 69, "D2": 73, "DS2": 78, "E2": 82, "F2": 87, "FS2": 93, "G2": 98, "GS2": 104, "A2": 110, "AS2": 117, "B2": 123, "C3": 131, "CS3": 139, "DB3": 139, "D3": 147, "DS3": 156, "EB3": 156, "E3": 165, "F3": 175, "FS3": 185, "G3": 196, "GS3": 208, "A3": 220, "AS3": 233, "B3": 247, "C4": 262, "CS4": 277, "D4": 294, "DS4": 311, "E4": 330, "F4": 349, "FS4": 370, "G4": 392, "GS4": 415, "A4": 440, "AS4": 466, "B4": 494, "C5": 523, "CS5": 554, "D5": 587, "DS5": 622, "E5": 659, "F5": 698, "FS5": 740, "G5": 784, "GS5": 831, "A5": 880, "AS5": 932, "B5": 988, "C6": 1047, "CS6": 1109, "D6": 1175, "DS6": 1245, "E6": 1319, "F6": 1397, "FS6": 1480, "G6": 1568, "GS6": 1661, "A6": 1760, "AS6": 1865, "B6": 1976, "C7": 2093, "CS7": 2217, "D7": 2349, "DS7": 2489, "E7": 2637, "F7": 2794, "FS7": 2960, "G7": 3136, "GS7": 3322, "A7": 3520, "AS7": 3729, "B7": 3951, "C8": 4186, "CS8": 4435, "D8": 4699, "DS8": 4978, "REST": 0 } def buzzer(note): buzzerPin.init() buzzerPin.freq(note) time.sleep_ms(500) buzzerPin.deinit() while True: distance = getSonar() time.sleep_ms(300) if distance: print(distance) if distance < 3: print('Do') ledPin.duty(125) buzzer(NOTES['A3']) elif distance >= 3 and distance < 7: #1 print('Re') ledPin.duty(250) buzzer(NOTES['B3']) elif distance >= 7 and distance < 10: #1 print('Mi') ledPin.duty(375) buzzer(NOTES['C3']) elif distance >= 10 and distance < 13: #2 print('Fa') ledPin.duty(500) buzzer(NOTES['D3']) elif distance == 13 and distance < 16: #3 print('So') ledPin.duty(625) buzzer(NOTES['E3']) elif distance >= 16 and distance < 20: #3 print('La') ledPin.duty(750) buzzer(NOTES['F3']) elif distance >= 20 and distance < 25: #3 print('Ti') ledPin.duty(875) buzzer(NOTES['G3']) buzzerPin.deinit()
Youtube video ↓ (click on it)
Project 2 - Infrared remote and components to turn on
Date: 11/2/23
Instructions:
Design and construct a circuit with an infrared remote, a buzzer, a motor (DC motor, servo motor, or stepper motor), a NeoPixel, and an LCD1602. By pressing various buttons on the infrared remote, their corresponding electronic components respond with some sound, spinning, light LEDs, or a message on the display. Capture your settings and working circuit in photos and videos (the videos should include sound).
Parts:
- ESP32-WROVER x1
- GPIO Extension Board x1 (optional)
- Breadboard x1
- LCD 1602 x1
- Jumper M/M x19
- Servo x1
- Neopixel 1x
- NPN transistor x1
- Passive Buzzer x7
- Thermistor x1
- Resistor 1kΩ x2
- Resistor 10kΩ x1
Infrared remote and components to turn on schema:
Infrared remote and components to turn on Breadboard View:
Source Code:
project-2.py
from machine import Pin, I2C, PWM import time from lcd import I2C, I2cLcd from infrared import irGetCMD from song import playSong from neop import neoRun from myservo import myServo servo=myServo(19) #set servo pin servo.myServoWriteAngle(0) #Set Servo Angle time.sleep_ms(1000) i2c = I2C(scl=Pin(33), sda=Pin(32), freq=400000) devices = i2c.scan() if len(devices) == 0: print("No i2c device !") else: for device in devices: print("I2C addr: "+hex(device)) lcd = I2cLcd(i2c, device, 2, 16) ############################################################# # Project Code ############################################################# ledPin=PWM(Pin(25),10000) # LED recvPin = irGetCMD(15) # Remote passiveBuzzer = PWM(Pin(13), 2000) # buzzer ledPin.duty(1) passiveBuzzer.deinit() while True: value = recvPin.ir_read() if value: if value == '0xff6897': #Message print('0') lcd.putstr("Made by \nAdam Boucek") time.sleep_ms(2000) lcd.putstr("") elif value == '0xff30cf': #LED print('1') ledPin.duty(1000) elif value == '0xff18e7': #Song print('2') playSong(passiveBuzzer) elif value == '0xff7a85': #Servo print('3') for i in range(0,180,1): servo.myServoWriteAngle(i) time.sleep_ms(15) for i in range(180,0,-1): servo.myServoWriteAngle(i) time.sleep_ms(15) elif value == '0xff10ef': #NEOPixel print('4') neoRun()
Youtube video ↓ (click on it)
Tutorials
To move forward I can show you what cool things you can make with the Freenove tutorials. They are well-guided and a lot of fun.
Tutorial No. | Gif |
---|---|
21.1 | ![]() |
21.2 | ![]() |
22.1 | ![]() |
22.2 | ![]() |
23.1 | ![]() |
23.2 | ![]() |
24.1 | ![]() |
24.2 | ![]() |